Code
library(DBI)
library(RSQLite)
library(connections)
Tony Duan
newColName mpg cyl disp hp drat wt qsec vs am gear carb
1 Mazda RX4 21.0 6 160 110 3.90 2.620 16.46 0 1 4 4
2 Mazda RX4 Wag 21.0 6 160 110 3.90 2.875 17.02 0 1 4 4
3 Datsun 710 22.8 4 108 93 3.85 2.320 18.61 1 1 4 1
4 Hornet 4 Drive 21.4 6 258 110 3.08 3.215 19.44 1 0 3 1
5 Hornet Sportabout 18.7 8 360 175 3.15 3.440 17.02 0 0 3 2
6 Valiant 18.1 6 225 105 2.76 3.460 20.22 1 0 3 1
newColName mpg cyl disp hp drat wt qsec vs am gear carb
1 Mazda RX4 21.0 6 160 110 3.90 2.620 16.46 0 1 4 4
2 Mazda RX4 Wag 21.0 6 160 110 3.90 2.875 17.02 0 1 4 4
3 Hornet 4 Drive 21.4 6 258 110 3.08 3.215 19.44 1 0 3 1
4 Hornet Sportabout 18.7 8 360 175 3.15 3.440 17.02 0 0 3 2
5 Valiant 18.1 6 225 105 2.76 3.460 20.22 1 0 3 1
6 Duster 360 14.3 8 360 245 3.21 3.570 15.84 0 0 3 4
newColName mpg cyl disp hp drat wt qsec vs am gear carb
1 Valiant 18.1 6 225 105 2.76 3.46 20.22 1 0 3 1
2 Cadillac Fleetwood 10.4 8 472 205 2.93 5.25 17.98 0 0 3 4
3 Dodge Challenger 15.5 8 318 150 2.76 3.52 16.87 0 0 3 2
newColName mpg cyl disp hp drat wt qsec vs am gear carb
1 Mazda RX4 21.0 6 160 110 3.90 2.620 16.46 0 1 4 4
2 Mazda RX4 Wag 21.0 6 160 110 3.90 2.875 17.02 0 1 4 4
3 Hornet 4 Drive 21.4 6 258 110 3.08 3.215 19.44 1 0 3 1
4 Hornet Sportabout 18.7 8 360 175 3.15 3.440 17.02 0 0 3 2
5 Valiant 18.1 6 225 105 2.76 3.460 20.22 1 0 3 1
6 Duster 360 14.3 8 360 245 3.21 3.570 15.84 0 0 3 4
newColName mpg cyl disp hp drat wt qsec vs am gear carb
1 Mazda RX4 21.0 6 160 110 3.90 2.620 16.46 0 1 4 4
2 Mazda RX4 Wag 21.0 6 160 110 3.90 2.875 17.02 0 1 4 4
3 Datsun 710 22.8 4 108 93 3.85 2.320 18.61 1 1 4 1
4 Hornet 4 Drive 21.4 6 258 110 3.08 3.215 19.44 1 0 3 1
5 Hornet Sportabout 18.7 8 360 175 3.15 3.440 17.02 0 0 3 2
6 Valiant 18.1 6 225 105 2.76 3.460 20.22 1 0 3 1
newColName mpg cyl disp hp drat wt qsec vs am gear carb
1 Cadillac Fleetwood 10.4 8 472 205 2.93 5.250 17.98 0 0 3 4
2 Lincoln Continental 10.4 8 460 215 3.00 5.424 17.82 0 0 3 4
3 Camaro Z28 13.3 8 350 245 3.73 3.840 15.41 0 0 3 4
4 Duster 360 14.3 8 360 245 3.21 3.570 15.84 0 0 3 4
5 Chrysler Imperial 14.7 8 440 230 3.23 5.345 17.42 0 0 3 4
6 Maserati Bora 15.0 8 301 335 3.54 3.570 14.60 0 1 5 8
newColName mpg cyl disp hp drat wt qsec vs am gear carb
1 Toyota Corolla 33.9 4 71.1 65 4.22 1.835 19.90 1 1 4 1
2 Fiat 128 32.4 4 78.7 66 4.08 2.200 19.47 1 1 4 1
3 Honda Civic 30.4 4 75.7 52 4.93 1.615 18.52 1 1 4 2
4 Lotus Europa 30.4 4 95.1 113 3.77 1.513 16.90 1 1 5 2
5 Fiat X1-9 27.3 4 79.0 66 4.08 1.935 18.90 1 1 4 1
6 Porsche 914-2 26.0 4 120.3 91 4.43 2.140 16.70 0 1 5 2
newColName mpg cyl disp hp drat wt qsec vs am gear carb
1 Cadillac Fleetwood 10.4 8 472 205 2.93 5.250 17.98 0 0 3 4
2 Lincoln Continental 10.4 8 460 215 3.00 5.424 17.82 0 0 3 4
3 Camaro Z28 13.3 8 350 245 3.73 3.840 15.41 0 0 3 4
4 Duster 360 14.3 8 360 245 3.21 3.570 15.84 0 0 3 4
5 Chrysler Imperial 14.7 8 440 230 3.23 5.345 17.42 0 0 3 4
6 Maserati Bora 15.0 8 301 335 3.54 3.570 14.60 0 1 5 8
newColName mpg new_mpg
1 Mazda RX4 21.0 21.0
2 Mazda RX4 Wag 21.0 21.0
3 Datsun 710 22.8 22.8
4 Hornet 4 Drive 21.4 21.4
5 Hornet Sportabout 18.7 18.7
6 Valiant 18.1 18.1
<SQLiteResult>
SQL create table if not exists new_mtcars as select * from mtcars order by mpg ,cyl
ROWS Fetched: 0 [complete]
Changed: 0
[1] "iris" "mtcars" "new_mtcars"
---
title: "SQL database with R"
author: "Tony Duan"
execute:
warning: false
error: false
format:
html:
toc: true
toc-location: right
code-fold: show
code-tools: true
number-sections: true
code-block-bg: true
code-block-border-left: "#31BAE9"
---
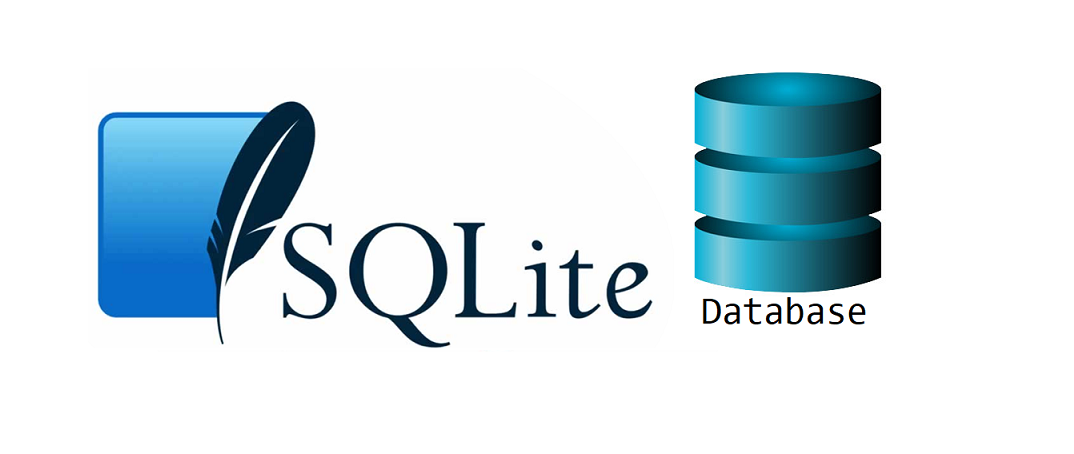{width="600"}
# Connection with database
```{r}
library(DBI)
library(RSQLite)
library(connections)
```
## create database file pythonsqlite.db and copy mtcars data and iris data into database
```{r}
mtcars=cbind(newColName = rownames(mtcars), mtcars)
```
```{r}
# Create an ephemeral in-memory RSQLite database
con <- dbConnect(RSQLite::SQLite(), "my_sql_database")
# view database on IDE
connection_view(con)
```
```{r}
#| eval: false
dbWriteTable(con, "mtcars", mtcars,overwrite=TRUE)
dbWriteTable(con, "iris", iris,overwrite=TRUE)
```
## check all table in database
```{r}
dbListTables(con)
```
# SQL
```{r}
data=dbReadTable(con, "mtcars")
head(data)
```
## select
```{r}
sql ="SELECT * FROM mtcars LIMIT 3"
table=dbGetQuery(con,sql)
table
```
## Renaming column
```{r}
sql="select mpg as new_mpg from mtcars"
table=dbGetQuery(con,sql)
head(table)
```
## create column
```{r}
sql="select mpg+1 as new_mpg,mpg from mtcars"
table=dbGetQuery(con,sql)
head(table)
```
## Filter rows
```{r}
sql="select * from mtcars where hp>100"
table=dbGetQuery(con,sql)
head(table)
```
### Filters with AND conditions
```{r}
sql="select * from mtcars where hp>100 and drat<3"
table=dbGetQuery(con,sql)
head(table)
```
### Filters with or conditions
```{r}
sql="select * from mtcars where hp>100 or drat<3"
table=dbGetQuery(con,sql)
head(table)
```
## Append
### append by row
```{r}
sql="select * from mtcars union all select * from mtcars "
table=dbGetQuery(con,sql)
head(table)
```
### append by column
### Dropping NA values
### keep NA values
## group by
### average,min,max,sum
```{r}
sql="select AVG(hp),min(hp),max(hp),sum(hp) from mtcars"
table=dbGetQuery(con,sql)
head(table)
```
### count record and count distinct record
```{r}
sql="select vs, count(*),count(distinct cyl) from mtcars group by vs"
table=dbGetQuery(con,sql)
head(table)
```
## order rows
```{r}
sql="select * from mtcars order by mpg"
table=dbGetQuery(con,sql)
head(table)
```
### Sort in descending order
```{r}
sql="select * from mtcars order by mpg desc"
table=dbGetQuery(con,sql)
head(table)
```
### Arrange by multiple variables
```{r}
sql="select * from mtcars order by mpg ,cyl"
table=dbGetQuery(con,sql)
head(table)
```
## join
### inner_join
```{r}
sql="select a.newColName,a.mpg,b.mpg as new_mpg from mtcars a left join mtcars b on a.newColName=b.newColName"
table=dbGetQuery(con,sql)
head(table)
```
### full join
### left join
### anti join
## Reshape tables
### Gather data long(wide to long)
### Spread data wide (long to wide)
## string
### upper case
### lower case
### match
### concatenation
### replace
### extract
## date
## create table into database
```{r}
sql="create table if not exists new_mtcars as select * from mtcars order by mpg ,cyl"
dbSendQuery(con,sql)
dbListTables(con)
```
## delete table in database
```{R}
sql="drop table if exists new_mtcars"
dbSendQuery(con,sql)
dbListTables(con)
```
## edit table in database
# Using SQL with R dataframe
```{R}
library(sqldf)
```
```{R}
data=sqldf("select * from mtcars LIMIT 3;")
data
```
# reference: